In previous article I had discuss about how we can use jquery dataTable plugin in javascript. So today in this article I am going to perform the full crud operation with localStorage in javascript. In this crud operation I am going to use some extra plugins for looking our operations more interactive and beautiful. And of course if we talking about giving beauty to our code then how we can ignore the bootstrap. So we are going to use bootstrap for our stylesheet and try to use very minimal css. So lets start the crud operation with localStorage in javascript.
Introduction to CRUD operation
CRUD stands for Create, Read, Update, and Delete—these are the basic operations that can be performed on data. In the context of JavaScript, CRUD operations are often associated with manipulating data in web applications or databases. Here’s a brief overview of how you might perform CRUD operations in JavaScript:
- Create : To create new data, you typically use the
POST
method in HTTP requests when working with a server. - Read: Reading data is typically done with the
GET
method in HTTP requests. - Update: To update data, you usually use the
PUT
orPATCH
methods in HTTP requests. - Delete: To delete data, you use the
DELETE
method in HTTP requests.
CRUD operation with localStorage
After Understanding CRUD operation lets go into more depth with doing crud operation with localStorage. If you don’t know about the localStorage then I will tell you shortly till then I will tell you that localStorage is place where we can store our data. On every browser there is localStorage in the application tab when you open inspect window.
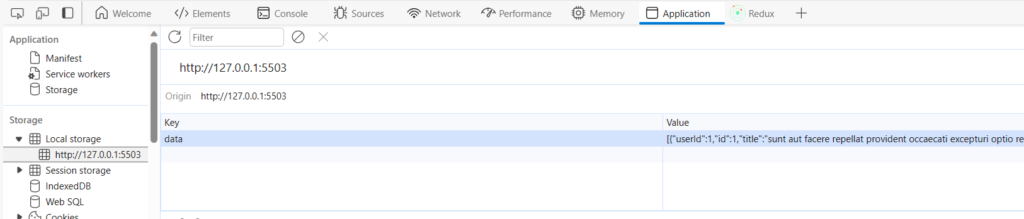
Above is the image of localStorage where we can store the data when we get the data from api. If we want to perform CRUD operation then definitely we have to store our data in either database or localStorage so when we refresh the page our data does not change. Like in database we can directly change data in database so on next time when we retreive the data i get the updated data. Same like database we are storing our data in localStorage and our every operation is performed through localStorage so that we can get the updated data. LocalStorage store the data in the form of key,value pair. Therefore you can see in key data we have stored lots of values.
Plugins used in CRUD operation with localStorage
In this CRUD operation with localStorage I have used three plugins which is used for searching, pagination , validation and showing notifications on every operation.
- DataTable Plugin : DataTable plugin is used for searching and pagination in the table.
- JQueryValidation Plugin : JQueryValidation Plugin is used for the validations of forms.
- Toast Plugin : Toast Plugin is used for showing notification when every operation is performed.
CRUD operation with localStorage in javascript Example
In this CRUD operation with localStorage in javascript I have made three files for different-2 code. For html I have index.html, for javascript I have script2.js and for style I have made style.css. Instead of this I have included all the CDNs in the index.html for all the plugins and bootstrap.
Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Bootstrap css cdn -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.0.0/dist/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous">
<!-- Jquery cdn link -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script>
<!-- Toast plugin cdn -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery-toast-plugin/1.3.2/jquery.toast.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.0/css/all.min.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery-toast-plugin/1.3.2/jquery.toast.min.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.0/css/all.min.css">
<!-- Jquery data table plugin -->
<link rel="stylesheet" href="//cdn.datatables.net/1.13.3/css/jquery.dataTables.min.css">
<link rel="stylesheet" href="style.css">
<title>Full crud operation with localStorage in javascript</title>
</head>
<body>
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#exampleModal" onclick="valid()">Add</button>
<!-- add Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Add New Data</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form action="" id="myform" onsubmit="return false">
<label for="title">Enter your Title :</label>
<input type="text" id="title" name="title" required><br><br>
<label for="body">Enter your Body :</label>
<input type="text" id="body" name="body" required><br><br>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary" id="set" onclick="addData()">Add</button>
</div>
</form>
</div>
</div>
</div>
</div>
<!-- edit modal -->
<div class="modal fade" id="editModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Edit Data</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form action="">
<label for="title1">Enter your Title :</label>
<input type="text" id="json_title" name="title1"><br><br>
<label for="body1">Enter your Body :</label>
<input type="text" id="json_body" name="body1"><br><br>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary" data-dismiss="modal" id="editchanges">Save changes</button>
</div>
</div>
</div>
</div>
<!-- details Modal -->
<div class="modal fade" id="detailModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Detail Of Data</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form action="">
<label for="userId">Your UserId :</label>
<input type="text" id="read_userid" name="userId"><br><br>
<label for="id">Your Id :</label>
<input type="text" id="read_id" name="id"><br><br>
<label for="title1">Your Title :</label><br>
<textarea name="title1" id="read_title" cols="30" rows="3"></textarea><br><br>
<label for="body1">Your Body :</label><br>
<textarea name="title1" id="read_body" cols="40" rows="5"></textarea><br><br>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<!-- displaying table -->
<p id="showdata"></p>
</body>
<!-- Toast Plugin cdn -->
<script src="script2.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-toast-plugin/1.3.2/jquery.toast.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-toast-plugin/1.3.2/jquery.toast.js"></script>
<!-- datatable plugin -->
<script src="https://cdn.datatables.net/1.13.3/js/jquery.dataTables.js"></script>
<!-- Bootstrap Cdn--------------------------------------------------------------Start -->
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.12.9/dist/umd/popper.min.js" integrity="sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.0.0/dist/js/bootstrap.min.js" integrity="sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/jquery-validation@1.19.5/dist/jquery.validate.js"></script>
<script src="https://cdn.jsdelivr.net/npm/jquery-validation@1.19.5/dist/jquery.validate.min.js"></script>
</html>
Style.css
#read_title,#read_body{
position: relative;
margin-left: 120px;
margin-top:-30px;
/* overflow: scroll;
background-color: aqua; */
}
.error{
color: red;
}
Script2.js
function getData()
{
const xml=new XMLHttpRequest();
xml.open("GET","https://jsonplaceholder.typicode.com/posts",true)
xml.onreadystatechange=function()
{
if(xml.readyState==4 && xml.status==200){
var js=JSON.parse(xml.responseText);
var v=localStorage.getItem("data")
if(v==""|| v==null){
localStorage.setItem("data",JSON.stringify(js))
createTable(js)
}
else{
createTable(JSON.parse(v))
}
}
}
xml.send();
}
getData();
function createTable(store){
let text="";
text=text+`<table border="1" class="table table-success table-striped" id="tabledata">
<thead>
<tr>
<th>UserId</th>
<th>Id</th>
<th>Title</th>
<th>Body</th>
<th>Operations</th>
</tr>
</thead>
<tbody>`
for(let i=0;i<store.length;i++){
text=text+`<tr>
<td>${store[i].userId}</td>
<td>${store[i].id}</td>
<td>${store[i].title}</td>
<td>${store[i].body}</td>
<td><button type="button" class="btn btn-primary btn-small" onclick="editdata(${store[i].id})"> Edit </button><br>
<button type="button" class="btn btn-small" onclick="details(${store[i].id})">Details</button><br>
<button type="button" class="btn btn-danger btn-small" onclick="deletedata(${store[i].id})" >Delete</button></td>
</tr>`;
}
text=text+`</tbody></table>`;
document.getElementById("showdata").innerHTML=text;
$('#tabledata').DataTable();
}
function valid(){
$("#myform").validate({
rules:{
}
})
}
function addData(){
let store = JSON.parse(localStorage.getItem("data"));
var length_store = store.length;
var newU = Math.floor((length_store/10)+1);
var newI = length_store+1;
var newT = $("#title").val();
var newB = $("#body").val();
var newOb = {
userId : newU,
id : newI,
title : newT,
body : newB,
};
store.push(newOb);
if(newT==""||newB==""){
valid();
return;
}
else
{
$.toast({
heading: 'Success',
text: 'Data Has Been Added Successfully',
showHideTransition: 'slide',
icon: 'success'
});
$("#exampleModal").modal('hide');
$(".modal-body input").val("")
}
localStorage.setItem("data", JSON.stringify(store));
document.getElementById("tabledata").innerHTML="";
createTable(store);
}
function deletedata(deleteindex){
let store=JSON.parse(localStorage.getItem("data"));
let index=deleteindex;
let result=confirm("Are you sure you want to delete this data");
if(result){
function findIndex(){
for(let i=0;i<store.length;i++){
if(index==store[i].id){
return i;
}
}
}
var d=findIndex();
store.splice(d,1);
$.toast({
heading: 'Deleted',
text: 'Data Has Been Deleted Successfully',
showHideTransition: 'slide',
icon: 'error',
loaderBg: 'red',
});
}
localStorage.setItem("data",JSON.stringify(store));
document.getElementById("tabledata").innerHTMl="";
createTable(store);
}
function editdata(editindex){
$('#editModal').modal('show');
let store=JSON.parse(localStorage.getItem("data"));
let index=editindex;
function findIndex(){
for(let i=0;i<store.length;i++){
if(index==store[i].id){
return i;
}
}
}
var x= findIndex();
var title=store[x].title;
var body=store[x].body;
document.getElementById("json_title").value=title;
document.getElementById("json_body").value=body;
$("#editchanges").click(function(){
var new_title=document.getElementById("json_title").value;
var new_body=document.getElementById("json_body").value;
store[x].title=new_title;
store[x].body=new_body;
$.toast({
heading: 'Updated',
text: 'Data Has Been Updated Successfully',
showHideTransition: 'slide',
icon: 'information',
loaderBg: 'Yellow',
});
localStorage.setItem("data",JSON.stringify(store));
document.getElementById("tabledata").innerHTML="";
createTable(store);
})
}
function details(detailindex){
$('#detailModal').modal('show');
let store=JSON.parse(localStorage.getItem("data"));
let index=detailindex;
//debugger;
function findIndex(){
for(let i=0;i<store.length;i++){
if(index==store[i].id){
return i;
}
}
}
var x= findIndex();
var userid=store[x].userId;
var id=store[x].id;
var title=store[x].title;
var body=store[x].body;
document.getElementById("read_userid").value=userid;
document.getElementById("read_id").value=id;
document.getElementById("read_title").value=title;
document.getElementById("read_body").value=body;
localStorage.setItem("data",JSON.stringify(store));
document.getElementById("tabledata").innerHTML="";
createTable(store);
document.getElementById("read_userid").disabled=true;
document.getElementById("read_id").disabled=true;
document.getElementById("read_title").disabled=true;
document.getElementById("read_body").disabled=true;
}
Some Glimpses of CRUD operation with localStorage
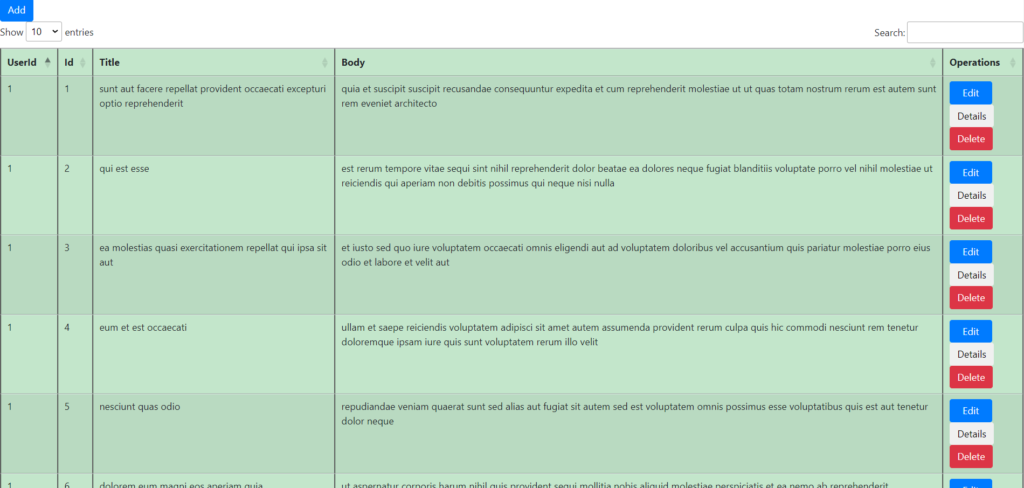
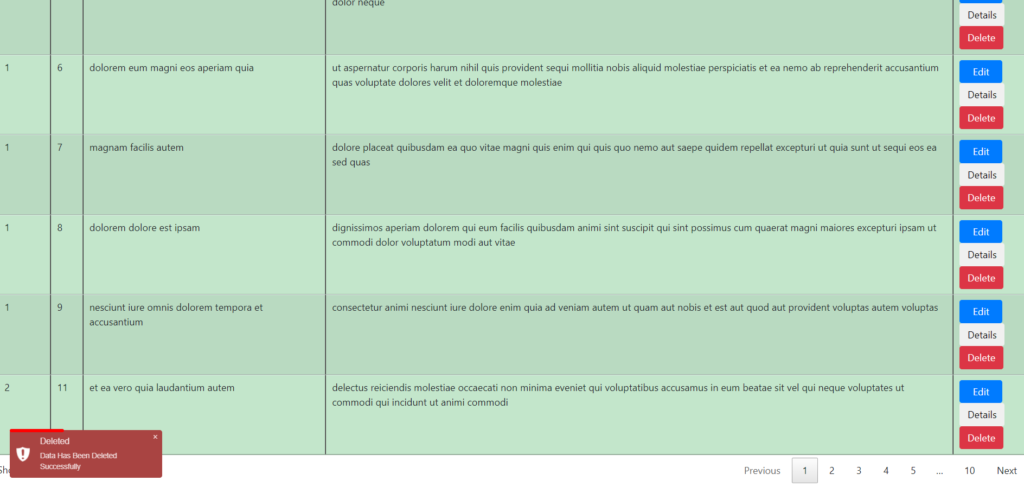
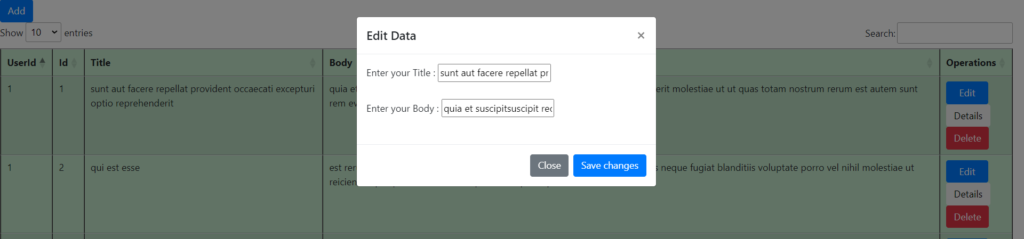
Source code
Above is the full code and some glimpses of how it works. You can see that it really looks very interative and also very user friendly by it looks. This is the whole crud operation some are might be thinking that it is very difficult to perform but it is all about the understanding sometime it takes time to understand but if you do this two three times then you feel confident to perform this. Now you can try this and I will also provide the zip file of source code.