In this article I am going to discuss about C# Continue Statement with example in detail but before that if you had not read my previous article of C# Break Statement then must read it for clear understanding. Continue Statement are not only used in csharp but also used in other programming language as well. It is one of the type of jump statement under control flow statements.
Introduction to C# Continue Statement
Like in C# Break Statement, break keyword is used to break the condition same like that in C# continue statement, continue keyword is used to skip the part of execution. In simple words continue statement is used to skip the current execution but not terminate the loop. For example, In a loop we have some condition which satisfies the loop’s condition but we does not want to execute the statement when this condition satisfies but we also want to execute the loop for rest of the condition so here if write break keyword then it will terminate the whole loop therefore to write this type of condition continue statement comes into existence. We can only skip that execution using C# continue statement. Let’s see the program to understand continue statement more clearly.
C# Program to Explain Continue Statement
using System; namespace ContinueStatementInCsharp { class Program { static void Main(string[] args) { for (int i = 1; i <= 5; i++) { if (i == 3) { continue; } Console.WriteLine("The Value of i is : "+i); } Console.ReadKey(); } } }
Output:
The Value of i is : 1
The Value of i is : 2
The Value of i is : 4
The Value of i is : 5
In above program we have simply write one for loop and inside for loop checking the condition that if i is equal to 3 then continue ( means skip this execution ). Therefore in output 3 is not printed but instead of continue keyword if we use break keyword then it will print the following output:
The Value of i is : 1
The Value of i is : 2
Continue Statement with Nested Loop
We can also use the continue statement with nested loops in csharp. For more clear understanding let see one small program that demonstrates the use of continue statement with nested loops.
using System; namespace NestedContinueStatements { class Program { static void Main(string[] args) { for (int i = 1; i <= 5; i++) { Console.WriteLine("The value of i is : "+i); for (int j = 1; j <= 5; j++) { if (j == 3) { continue; } Console.WriteLine(" The value of j is : "+j); } Console.WriteLine(); } Console.ReadKey(); } } }
Output:
The value of i is : 1
The value of j is : 1
The value of j is : 2
The value of j is : 4
The value of j is : 5
The value of i is : 2
The value of j is : 1
The value of j is : 2
The value of j is : 4
The value of j is : 5
The value of i is : 3
The value of j is : 1
The value of j is : 2
The value of j is : 4
The value of j is : 5
The value of i is : 4
The value of j is : 1
The value of j is : 2
The value of j is : 4
The value of j is : 5
The value of i is : 5
The value of j is : 1
The value of j is : 2
The value of j is : 4
The value of j is : 5
In above program I have simply write one loop and inside loop there is another loop then am checking the condition if i is equal to 3. If yes then am skiping this execution using continue keyword and rest is working as it is.
Difference between Break and Continue Statement
The difference between break and continue statement is discussed below:
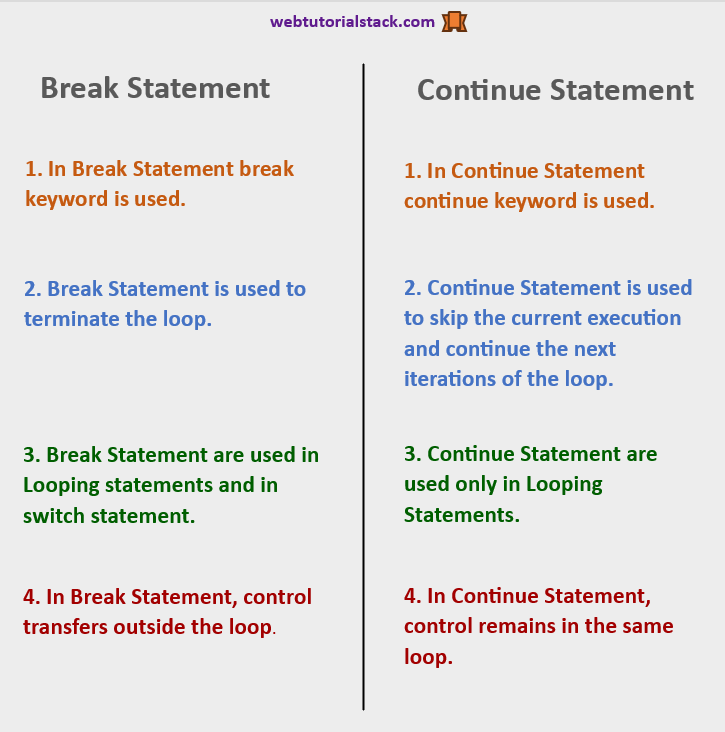
Conclusion
In this C# tutorials article I have discussed about continue statement with example in detail. I have also covered the difference between break and continue statement. Hope you liked this article and understand about the continue statement in csharp. In my next article I am going to discuss about goto statement with example in detail.