In this article I am going to discuss about C# command line arguments with example but before that if you had not read my previous article of C# recursion then must read it. You Everyone know that a C# program will contain one main method but have you ever tried to pass the arguments to this function. As a beginner most of them have not tried it and some might be thinking that can be do it? The answer is yes we can pass the parameters to our main function. So lets discuss it and know about command line arguments in csharp.
Introduction to C# Command Line Arguments
When we pass any parameter to our Main method of our C# program then it is considered as command line arguments. But this Main method will accept the parameters only in the form of string so we can only pass string to our Main method as command line arguments in C#. Remember main method does not accept any parameter from other methods but it will accept parameters as command line arguments. You must have noticed that C# main method contains the string[] args which means string array of arguments so we can only pass string as C# command line arguments.
How to Pass Command Line Arguments?
For passing Command Line arguments in your csharp program then create an console application and follow the following steps.
1. Create one Console application.
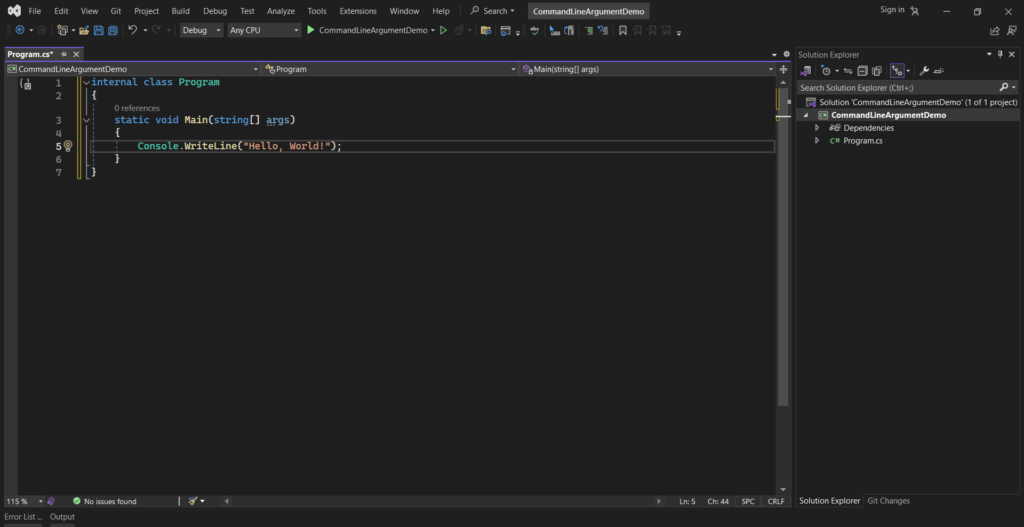
2. After creating console application right click on your application name( in my case CommandLineArgumentDemo).
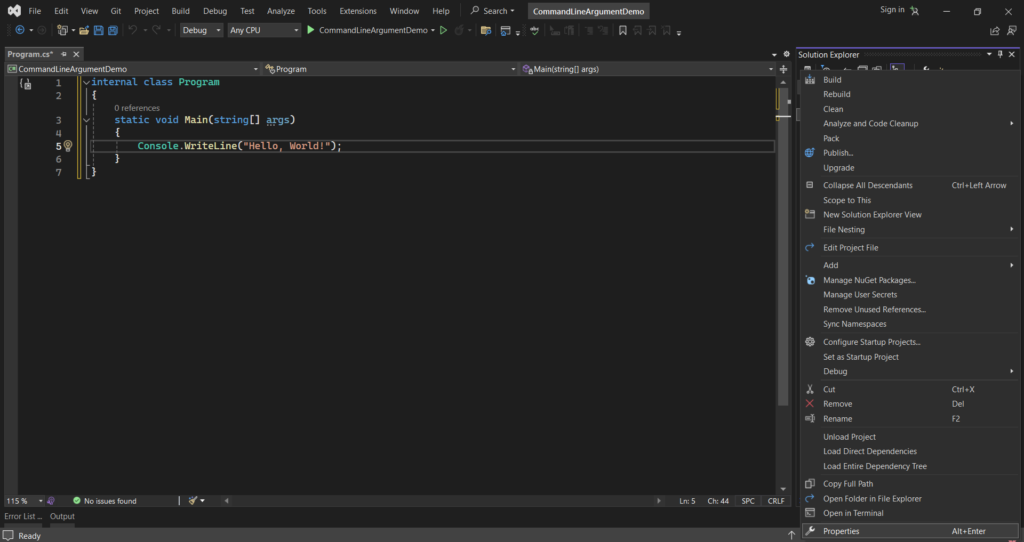
3. Now choose properties from the options.
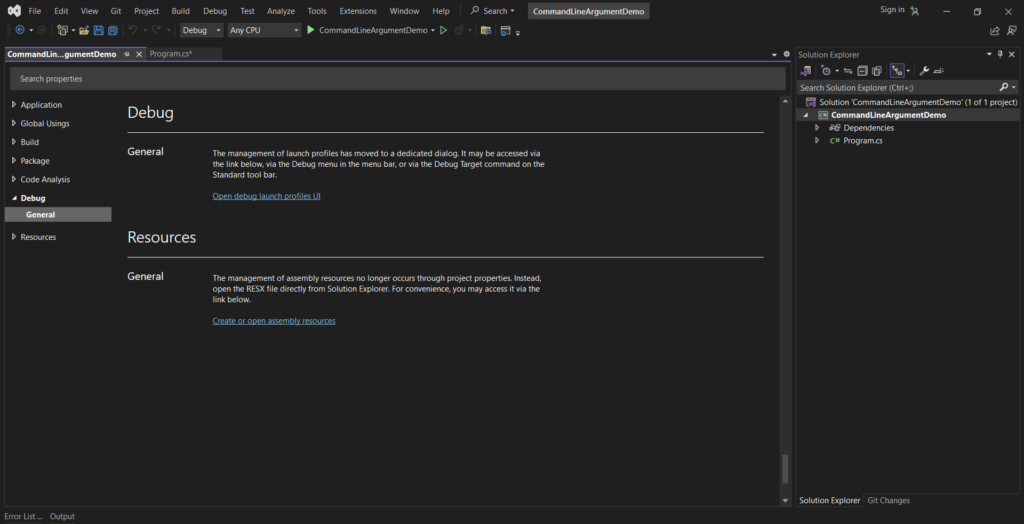
4. When you choose properties then above options are appear and you have to choose Debug-> General and click on open debug launch profiles UI.
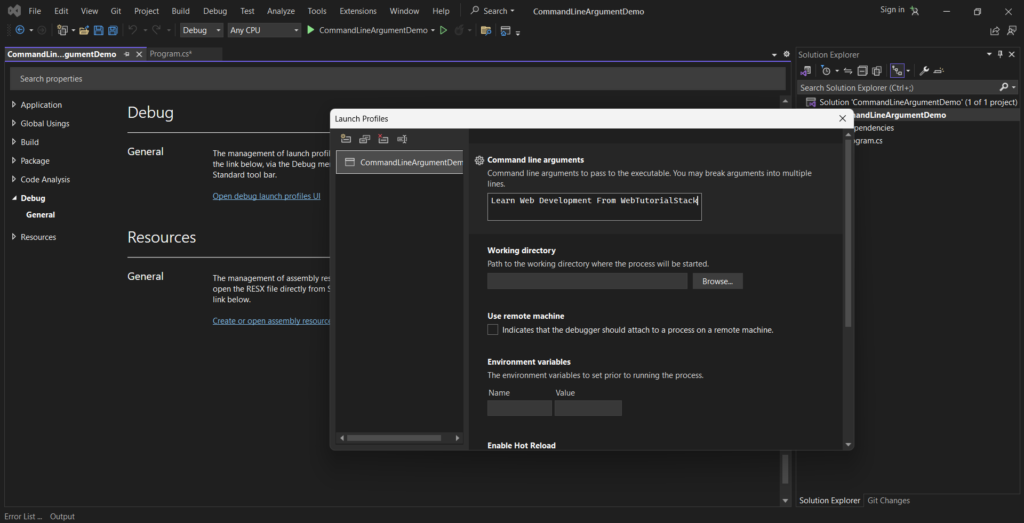
5. When you click on open debug launch profiles UI then the above launch profiles dialog will open and you have to fill the arguments(in my case Learn Web Development From WebTutorialStack). Remember it will take the parameters in the form of string array so pass accordingly.
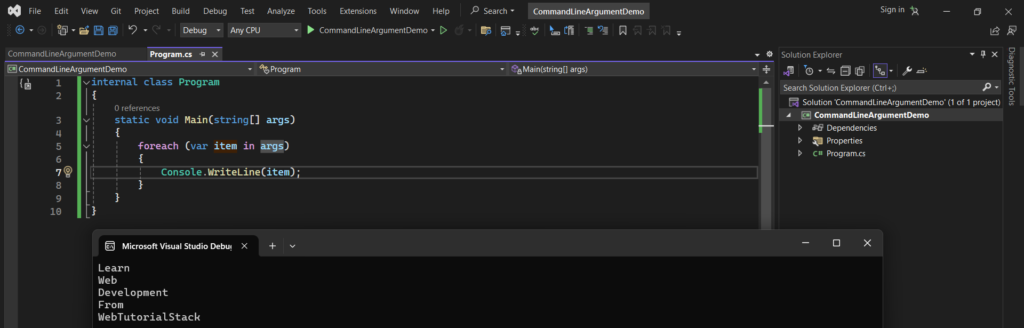
6. Modify your program as above and then run your application. you can see the command line arguments in the output window like in above image.
How to display number through Command Line Arguments?
If you want to display numbers from command line arguments then you have to pass the numbers in the form of string to command line arguments and then typecast the arguments in your program to display the numbers in your output screen. For e.g
using System; namespace FirstProgram { class Program { static void Main(string[] args) { foreach (var item in args) { Console.WriteLine(Convert.ToInt32(item)); } Console.ReadLine(); } } }
In above program if you have not passed the number in correct format of string then you might see exception here. So put valid values in the C# command line arguments screen.
Conclusion
In this C# tutorials article I have discussed the Command Line Arguments with example and also show how to give the command line arguments. Hope you found this article helpful and like this article. In my next article I am going to start the object oriented programming which is very very important if you want to become a good backend developer.