In this article I am going to discuss about C# Built-In Functions in detail but before that if you had not read my previous article of C# Functions then must read it for more clear understanding. In every programming language there are some predefined functions that is used for short our program and ease our work. Same, In C# Built-in functions are the functions which is already defined in the framework and available to be used by any programmer to do the task with few lines of code. There are lots of C# functions are there which is used by the programmer. So I’ll try to cover most of the C# functions with an example.
C# Built-In Math Functions
In C# built-in functions there is class named Math which provides different methods to perform mathematical calculations or perform specific task. For example we can find maximum of two number, minimum of two number, absolute number and so on. The mostly used C# built-in math functions are discussed below:
- Math.Max()
- Math.Min()
- Math.Sqrt()
- Math.Abs()
- Math.Round()
- Math.Floor()
- Math.Ceiling()
Math.Max( ) and Math.Min( )
In C# Built-in functions Math.Max() is used to find the greatest number and Math.Min() is used to find the smallest number.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { Console.WriteLine("The maximum of 6 and 7 is {0}", Math.Max(6,7)) Console.WriteLine("The maximum of 6 and 7 is {0}", Math.Min(6,7)); Console.ReadKey(); } } }
Output:
The maximum of 6 and 7 is 7
The minimum of 6 and 7 is 6
Math.Abs( )
In C#, Math.Abs() built-in function is used to find the absolute(positive) value any number.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { Console.WriteLine("The absolute value of -6 is {0}", Math.Abs(-6)); Console.ReadKey(); } } }
Output:
The absolute value of -6 is 6
Math.Sqrt( )
In C#, Math.Sqrt() function is used to find the square root of any number.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { Console.WriteLine("The square root of 9 is {0}", Math.Sqrt(9)); Console.ReadKey(); } } }
Output:
The square root of 9 is 3
Math.Round( ), Math.Floor( ) and Math.Ceiling( )
In C# Math.Round() function is used to find the round figure of any decimal value, Math.Floor() function is used to find the floor value of any number and Math.Ceiling() function is used to find the ceiling value of any number.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { Console.WriteLine("The Round Value of 7.6 is {0}",Math.Round(7.6)); Console.WriteLine("The Floor Value of 7.6 is {0}",Math.Floor(7.6)); Console.WriteLine("The Ceil Value of 7.6 is {0}",Math.Ceiling(7.6)); Console.ReadKey(); } } }
Output:
The Round Value of 7.6 is 8
The Floor Value of 7.6 is 7
The Ceil Value of 7.6 is 8
C# Built-In String Functions
Like Math Functions there are also some built-in string functions in C# which is used to manipulate the string. These functions are discussed below :
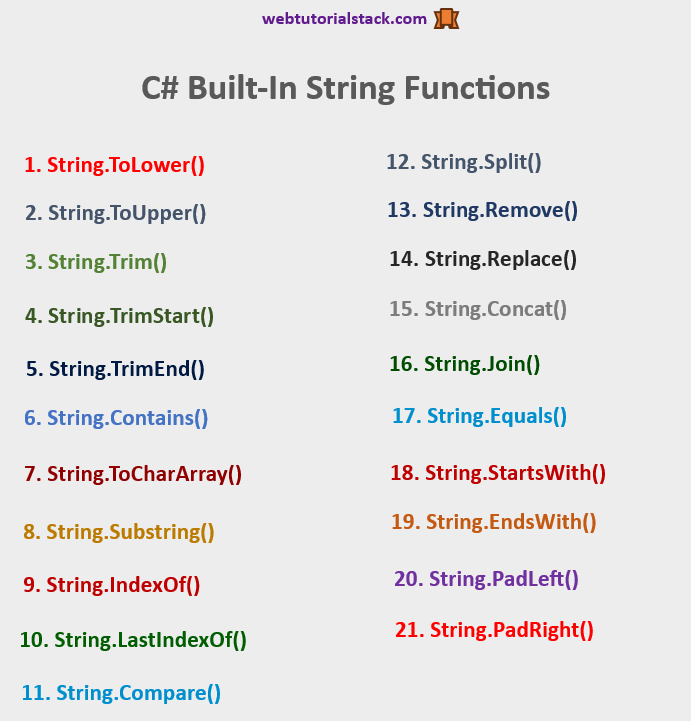
String.ToLower( ) and String.ToUpper( )
In C#, String.ToUpper() function is used to convert the string to upper case characters and String.ToLower() function is used to convert the string to lower case characters.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { string name="WEBTUTORIALSTACK.COM"; string name2="Learn full stack web development"; Console.WriteLine("Lower case of WEBTUTORIALSTACK.COM is : "+ name.ToLower()); Console.WriteLine("Upper case of {0} is : {1}", name2, name2.ToUpper()); Console.ReadKey(); } } }
Output:
Lower case of WEBTUTORIALSTACK.COM is : webtutorialstack.com
Upper case of Learn full stack web development is : LEARN FULL STACK WEB DEVELOPMENT
String.Trim( ), String.TrimStart( ) and String.TrimEnd( )
String.Trim() function is used to remove whitespaces from the starting and ending of the string, String.TrimStart() function is used to remove whitespaces from beginning of the string and String.TrimEnd() is used to remove whitespaces from ending of the string.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { string name=" WebTutorialStack "; Console.WriteLine("Remove whitespaces from string : "+ name.Trim()); Console.WriteLine("Remove whitespaces from begining of string : "+ name.TrimStart()); Console.WriteLine("Remove whitespaces from ending of string : "+ name.TrimEnd()); Console.ReadKey(); } } }
Output:
Remove whitespaces from string : WebTutorialStack
Remove whitespaces from begining of string : WebTutorialStack
Remove whitespaces from ending of string : WebTutorialStack
String.Contains( )
String.Contains() function is used to check whether the specified character or string is present in the string or not. If present then it returns true otherwise it will return false.
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { string name="WebTutorialStack.com is best place to learn web development"; bool check=name.Contains("webtutorialstack"); bool checkWithCaseSenstivity=name.Contains("WebTutorialStack"); Console.WriteLine("webtutorialstack is present : " +check); Console.WriteLine("WebTutorialStack is present : "+ checkWithCaseSenstivity); Console.ReadKey(); } } }
Output:
webtutorialstack is present : False
WebTutorialStack is present : True
As we know C# is case sensitive programming language therefore for the first time it has returned false and when we write exact string then it has returned true. But if you want to check the string with case sensitivity constraint then you can use StringComparison.OrdinalIgnoreCase and if you want to check the string with case sensitivity constraint then you can use StringComparison.Ordinal ( this is by default ). Like:
using System; namespace BuiltInFunction { class Program { static void Main(string[] args) { string name="WebTutorialStack.com is best place to learn web development"; bool check=name.Contains("webtutorialstack",StringComparison.Ordinal); bool checkWithCaseSenstivity=name.Contains("webtutorialstack",StringComparison.OrdinalIgnoreCase); Console.WriteLine("webtutorialstack is present : " +check); Console.WriteLine("webtutorialstack is present : "+ checkWithCaseSenstivity); Console.ReadKey(); } } }
Output:
webtutorialstack is present : False
webtutorialstack is present : True
String.ToCharArray( )
String.ToCharArray() method is used to convert the whole string into array of characters. But this method will also take some parameters to show the characters as per our choice. For example if we only want the characters from specified index then we can pass the index number and length of string from that particular index.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string str = "WebTutorialStack"; char[] result; int indexFromCharacterStart=3; int lengthOfString=4; // copies str to result result = str.ToCharArray(); // prints result for (int i = 0; i < result.Length; i++) { Console.WriteLine(result[i]); } Console.WriteLine("--------------------------------"); Console.WriteLine("Printing the string characters from specified index and specified length"); result= str.ToCharArray(indexFromCharacterStart,lengthOfString); for (int i = 0; i < result.Length; i++) { Console.WriteLine(result[i]); } Console.ReadLine(); } } }
Output:
W
e
b
T
u
t
o
r
i
a
l
S
t
a
c
k
--------------------------------
Printing the string characters from specified index and specified length
T
u
t
o
As you can see from above program then when we used ToCharArray() without passing any parameter then it has returned the result in form of char array but when we have specified the index and length then it has only returned the character which is starting from index 3 and we have specified the length to 4 therefore it has only printed 4 characters. Remember in C# string index is start from 0.
String.Substring( )
String.Substring() function is used to return the substring of the given string and will take either one parameter which is index from where substring starts or take two parameters where we specify the start index and length of substring.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string str = "WebTutorialStack"; Console.WriteLine("The Substring of {0} from index 1 is : {1}",str, str.Substring(1)); Console.WriteLine("The Substring of {0} from specified index and length is : {1}",str, str.Substring(1,7)); Console.ReadLine(); } } }
Output:
The Substring of WebTutorialStack from index 1 is : ebTutorialStack
The Substring of WebTutorialStack from specified index and length is : ebTutor
String.IndexOf( ) and String.LastIndexOf( )
String.IndexOf() function returns the index of given string and if string not found then it will return -1. String.LastIndexOf() function returns the last index of given string and if string not found then it will return -1. We can use these method with many ways like :
- Only passing one parameter which is either character or string ,
- With passing two parameters which is character and index this means that it will find the index of character from specified index. And
- With passing three parameters which is character , index and count this means theat it will find the index of character from specified index till the specified count. This will more clear with an example.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string str = "WebTutorialStack"; Console.WriteLine("The index of a is : {1}",str, str.IndexOf('a')); Console.WriteLine("The index of e from index 2 is : {1}",str, str.IndexOf('e',2)); Console.WriteLine("The index of e from index 2 and count 4 is : {1}",str, str.IndexOf('o',2,8)); //LastIndexOf Console.WriteLine("The last index of a is : {1}",str, str.LastIndexOf('a')); Console.WriteLine("The last index of e from index 2 is : {1}",str, str.LastIndexOf('e',2)); Console.WriteLine("The last index of W from index 7 and count 4 is : {1}",str, str.LastIndexOf('u',7,4)); Console.ReadLine(); } } }
Output:
The index of a is : 9
The index of e from index 2 is : -1
The index of e from index 2 and count 4 is : 6
The last index of a is : 13
The last index of e from index 2 is : 1
The last index of W from index 7 and count 4 is : 4
String.Split( ) and String.Remove( )
String.Split() function is used to split the string from the given character and String.Remove() function is used to remove the characters from specified index and it will also take second parameter to remove number of characters from specified index.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string str = "WebTutorialStack.com"; string[] breakMysentence = str.Split('.'); Console.WriteLine("Spliting the string from dot"); foreach (string data in breakMysentence){ Console.WriteLine(data); } //Remove Characters Console.WriteLine("Remove characters from index 3 : "+ str.Remove(3)); Console.WriteLine("Remove characters from index 3 : "+ str.Remove(3,4)); Console.ReadLine(); } } }
Output:
Spliting the string from dot
WebTutorialStack
com
Remove characters from index 3 : Web
Remove characters from index 3 : WebrialStack.com
String.Replace( ) and String.Concat( )
String.Replace() function is used to replace the given string with specified string. String.Concat() function is used to concatenates two strings.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string str = "WebTutorialStack.com"; //Replace the string Console.WriteLine("The WebTutorialStack.com has been replaced with : {0} ", str.Replace("WebTutorialStack.com","Make a career with web development")); Console.WriteLine("---------------------------"); //concating the string string string1="Sachin"; string string2="Tendulkar"; string[] stringArray={"Learn","Web","Development"}; Console.WriteLine("After concatenating two strings :" + String.Concat(string1,string2)); Console.WriteLine("After concatenating string array :" + String.Concat(stringArray)); Console.ReadLine(); } } }
Output:
The WebTutorialStack.com has been replaced with : Make a career with web development
---------------------------
After concatenating two strings :SachinTendulkar
After concatenating string array :LearnWebDevelopment
String.Join( )
String.Join() function is used to join two or more than two strings into one string. This function will take different-2 parameters to work with string. The parameters taken by String.Join() function is discussed below:
- separator – This is first parameter which is the separator to join the elements.
- value – This is second parameter which will take an array of strings to join.
- startIndex – This is the third parameter which will take the first item in value to join
- count – The fourth parameter which will take number of elements to join (starts from startIndex).
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string[] stringArray={"Learn","Web","Development"}; Console.WriteLine("After joining string Array :" + String.Join("-",stringArray)); Console.WriteLine("After joining string array with all parameters :" + String.Join("-",stringArray,1,2)); Console.ReadLine(); } } }
Output:
After joining string Array :Learn-Web-Development
After joining string array with all parameters :Web-Development
String.Equals( )
String.Equals() function is used to check whether two strings are same or not.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { // Checking Whether two strings are same or not string string1="WebTutorialStack"; string string2="webtutorialstack"; Console.WriteLine("Checking two strings are same or not :" + String.Equals(string1, string2)); Console.WriteLine("Checking two strings with ignoring case are same or not :" + String.Equals(string1, string2,StringComparison.OrdinalIgnoreCase)); Console.ReadLine(); } } }
Output:
Checking two strings are same or not :False
Checking two strings are same or not :True
String.StartsWith( ) and String.EndsWith( )
String.StartsWith() function is used to check whether the string is start with specified string or not. String.EndsWith() function is used to check whether the string is end with specified string or not.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string string1="WebTutorialStack"; string string2="webtutorialstack"; Console.WriteLine("String is starts with ignoring case Web :" + string1.StartsWith("web",StringComparison.OrdinalIgnoreCase)); Console.WriteLine("String is starts without ignoring case Web :" + string1.StartsWith("web")); Console.WriteLine("String is ends with Web :" + string1.EndsWith("Web")); Console.ReadLine(); } } }
Output:
String is starts with ignoring case Web :True
String is starts without ignoring case Web :False
String is ends with Web :False
String.PadLeft( ) and String.PadRight( )
In C# built-in functions there are String.PadLeft() and String.PadRight() functions are there which is used to give padding to our string with padding character.
using System; namespace BuiltInFunctions { class Program { static void Main(string [] args) { string string1="WebTutorialStack"; Console.WriteLine("Padding Left : " + string1.PadLeft(30)); Console.WriteLine("Padding Left : " + string1.PadLeft(30,'_')); Console.WriteLine("Padding Left : " + string1.PadRight(30,'_')); Console.ReadLine(); } } }
Output:
Padding Left : WebTutorialStack
Padding Left : ______________WebTutorialStack
Padding Left : WebTutorialStack______________
Other C# Built-In Functions
Instead of above these C# built-in functions there are some more built-in functions are there in csharp which we have already discussed in our previous articles so that’s why I am not discussing this methods here again. If you want to read about these methods then please read our please articles.
- ToString() : Which is used to convert the number to string.
- Convert.ToInt32(): Which is used to convert the string to number.
- Int32.Parse(): Which is also used to convert the string to number.
- Convert.ToDouble(): Which is used to convert the string to double value.
- Convert.ToDecimal(): Which is used to convert the string to decimal value.
Conclusion
In this C# tutorials articles I have discussed about C# built-in functions in very detail. I have covered almost every mostly used built-in functions in C#. In addition to this I have write programs for each and every function so that it is clear to you very easily. In my next article I am going to discuss about Call by reference and call by value in C# which is again the important topic in csharp programming language. Hope you like this article and understand the C# built-in functions.