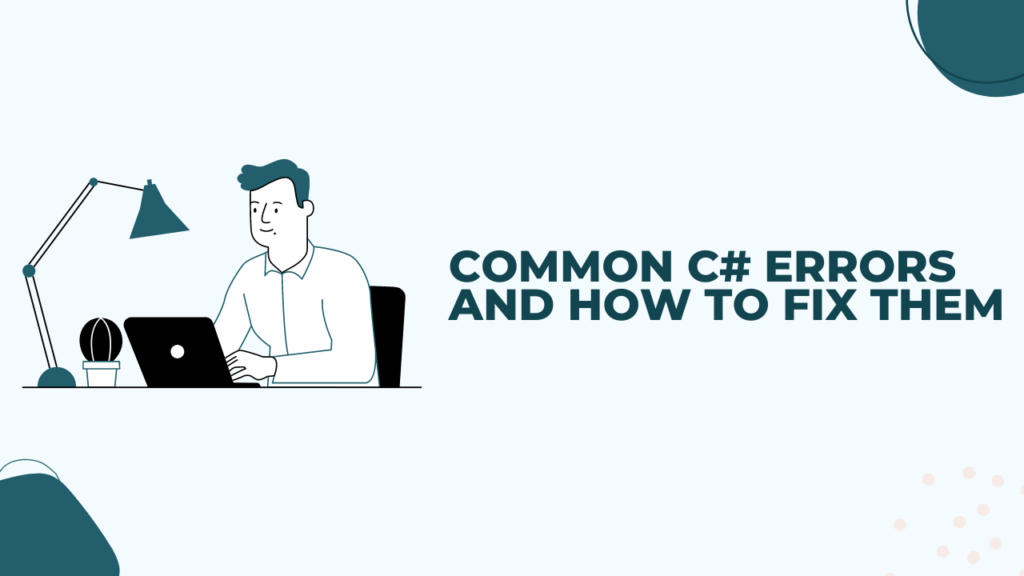
C# (C-Sharp) is a powerful and versatile programming language that has become increasingly popular in the software development community. As with any programming language, there are common mistakes that developers often make when working with C#. These mistakes can lead to bugs, performance issues, and other problems that can be difficult to diagnose and fix. In this article, we will explore some of the most common C# mistakes and provide practical tips on how to avoid them.
Avoid These Common C# Mistakes
1. Ignoring Null Checks:- One of the most common C# mistake is ignoring null checks. In C#, checking for null values before accessing an object’s properties or methods is essential. Failing to do so can lead to a NullReferenceException, which can cause your application to crash. To avoid this, always remember to perform null checks before accessing any object properties or methods.
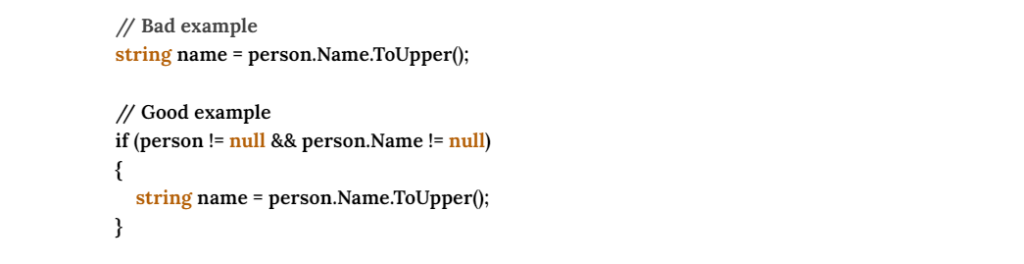
2. Improper Exception Handling:- Proper exception handling is crucial in C# development. Catching and handling exceptions correctly can help you provide a better user experience and prevent your application from crashing. However, many developers make the mistake of either not handling exceptions at all or catching too broad of an exception type, which can lead to unintended consequences.
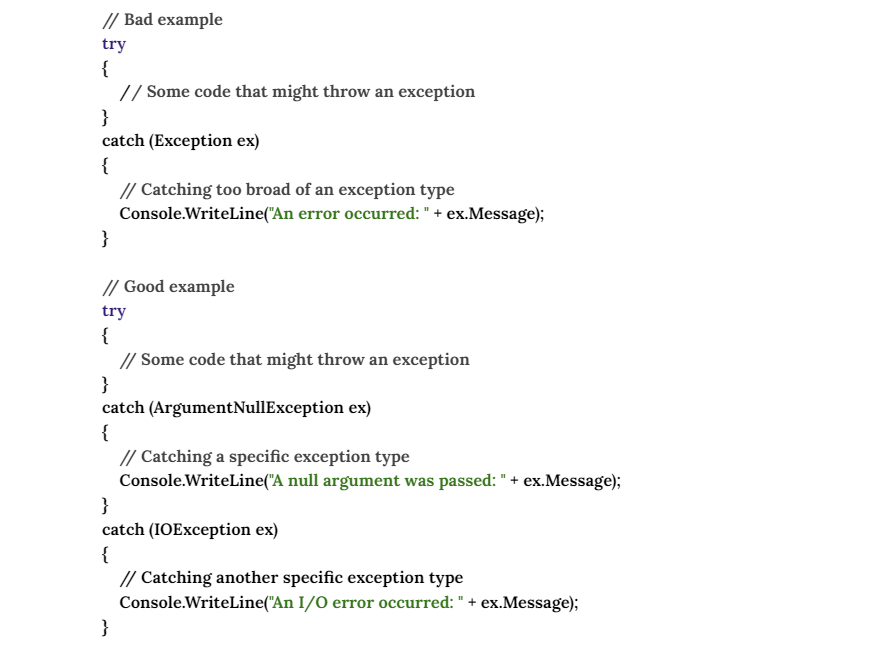
3. Inefficient Use of LINQ:- LINQ (Language Integrated Query) is a powerful feature in C# that allows you to perform complex data manipulations in a concise and readable way. However, some developers may not fully understand how to use LINQ efficiently, leading to performance issues or unexpected results.
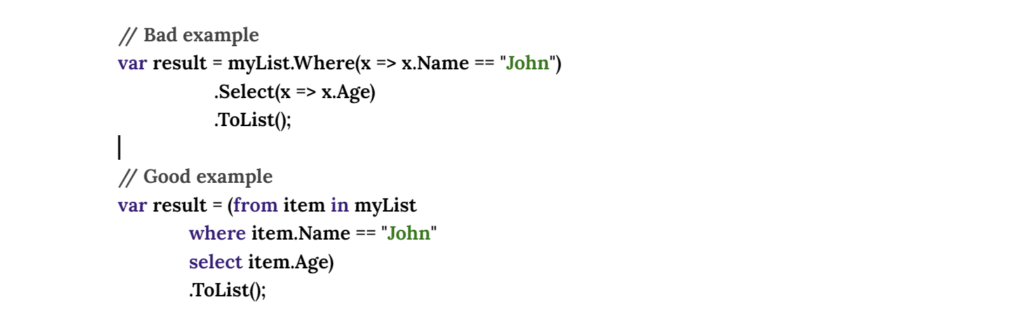
4. Misunderstanding Asynchronous Programming:- Asynchronous programming is a crucial concept in modern C# development, as it allows your application to perform long-running tasks without blocking the main thread. However, many developers struggle to understand the proper use of async and await keywords, leading to deadlocks, race conditions, and other concurrency-related issues.
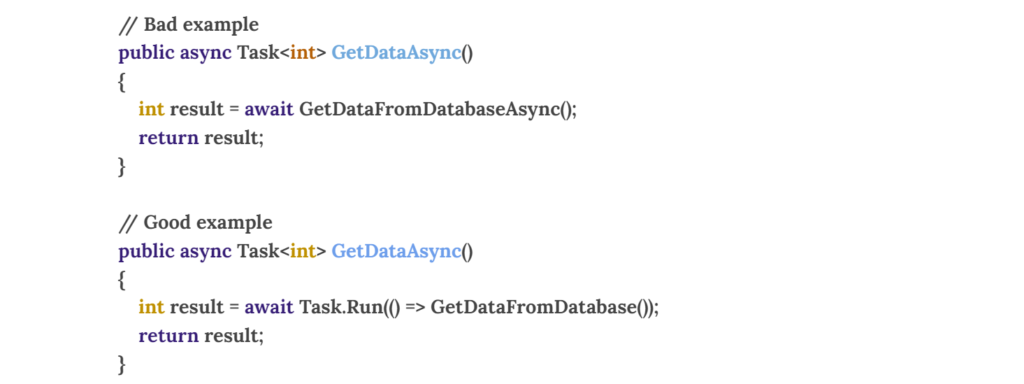
5. Forgetting to Dispose of Resources:- In C#, it’s important to properly dispose of resources, such as database connections, file handles, and network sockets, to avoid resource leaks and ensure your application’s stability. Failing to do so can lead to performance issues and even crashes.
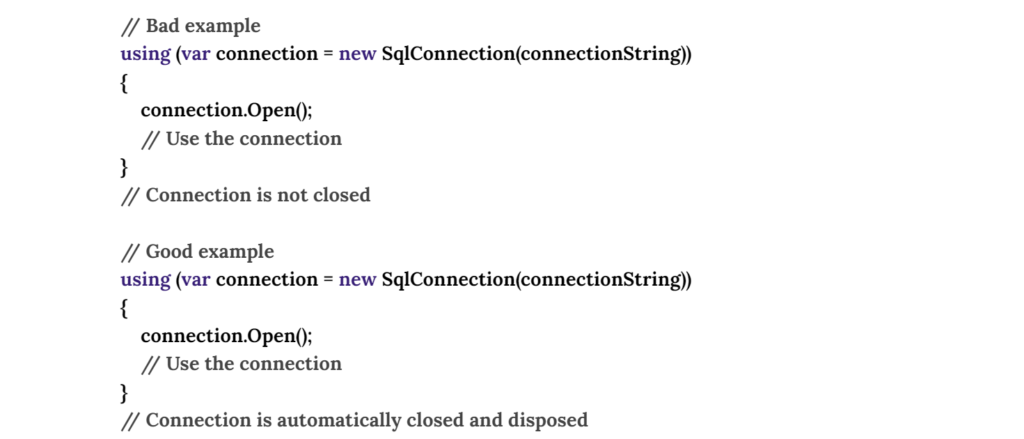
6. Neglecting Code Optimization:- C# is a powerful language, but it’s important to write efficient and optimized code to ensure your application’s performance. Neglecting code optimization can lead to slow response times, high resource usage, and other performance-related issues.
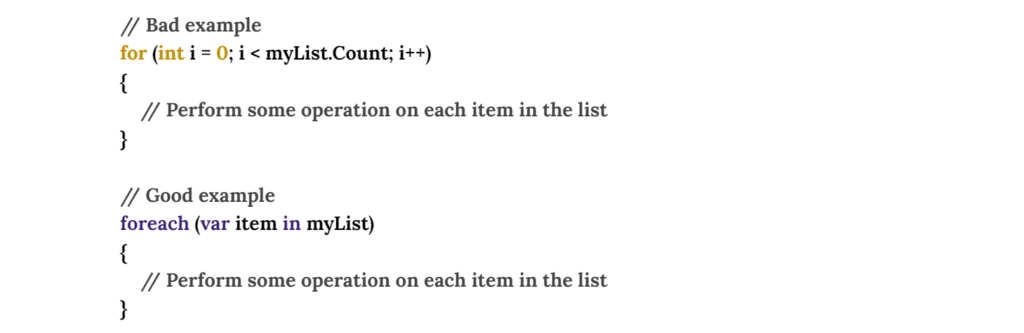
7. Improper Use of Generics:- Generics are a powerful feature in C# that allow you to write type-safe code and reuse code across different data types. However, some developers may not fully understand how to use generics correctly, leading to type-related errors and other issues.
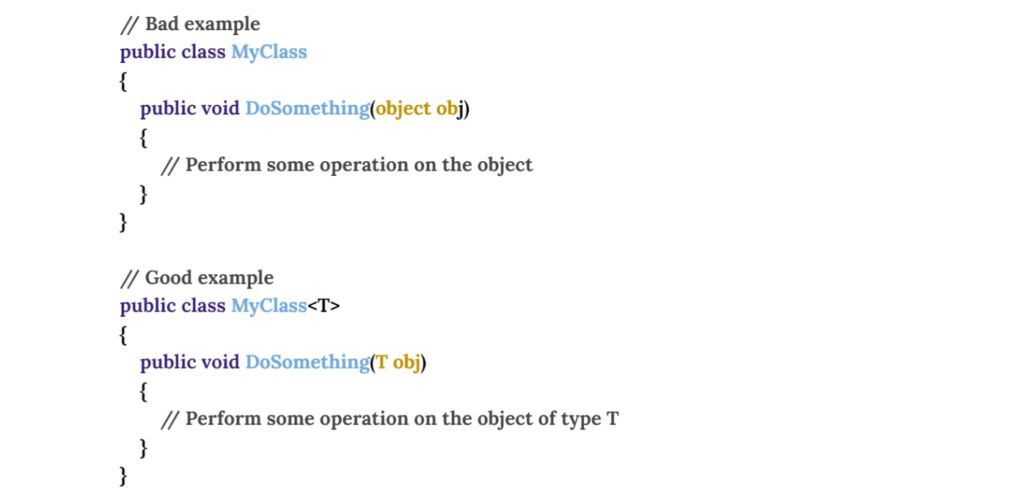
8. Failing to Implement Proper Logging:- Logging is a crucial component in C# applications, enabling developers to monitor and troubleshoot runtime issues. Failing to implement proper logging can make it difficult to troubleshoot and debug your application.
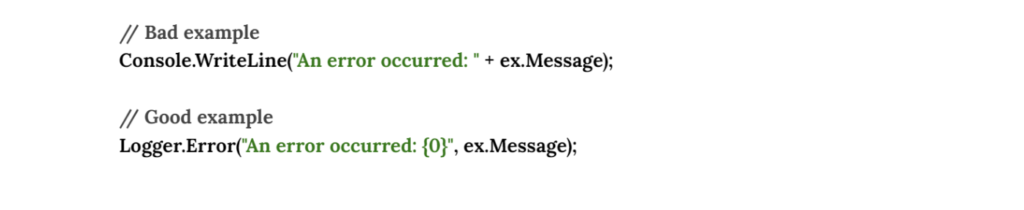
9. Neglecting Unit Testing:- Unit testing is a crucial practice in software development, as it helps you catch bugs early, ensure code quality, and facilitate refactoring. Neglecting unit testing can lead to a fragile codebase and make it more difficult to maintain and extend your application.
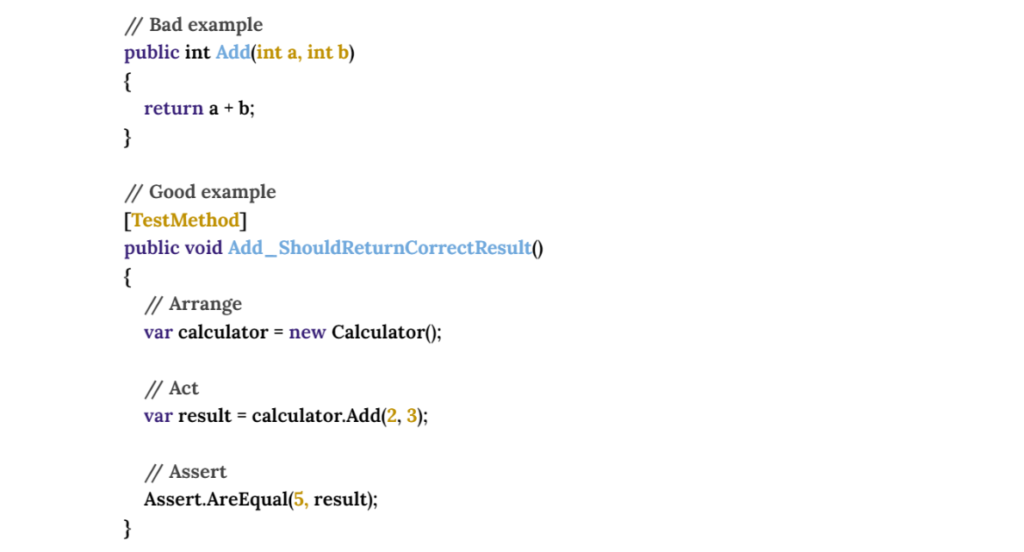
10. Ignoring Security Best Practices:- Security is a critical concern in any C# application, and it’s important to follow best practices to protect your application and its users from potential threats. Ignoring security best practices can lead to vulnerabilities that can be exploited by malicious actors.
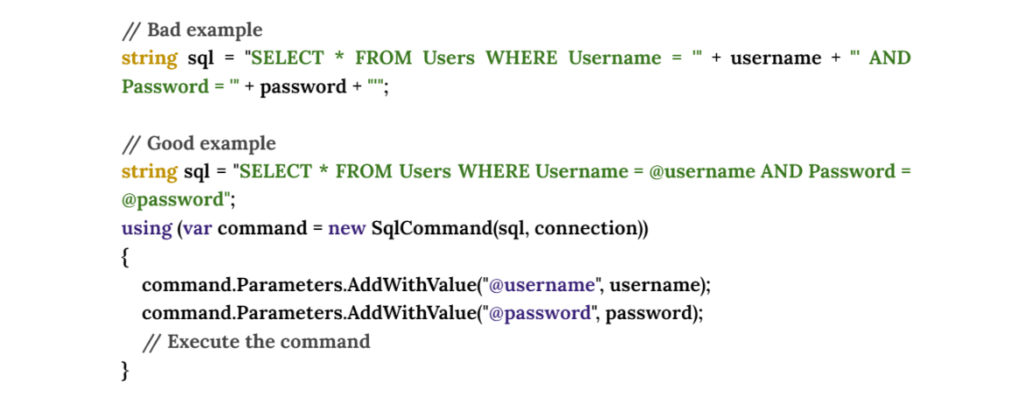
Conclusion
In this article, we’ve explored some of the most common C# mistakes and provided practical tips on how to avoid them. By understanding these common pitfalls and following best practices, you can write more robust, efficient, and secure C# code. Remember, the key to avoiding these mistakes is to stay vigilant, continuously learn, and always strive to write clean, maintainable, and high-performing C# applications.
FAQs
What is the most common C# mistake, and how can I avoid it?
The most common C# the mistake is ignoring null checks. To avoid this, always remember to perform null checks before accessing any object properties or methods.
How can I improve my exception handling in C#?
To improve your exception handling in C#, be sure to catch and handle specific exception types, rather than catching a broad Exception type. This will help you provide more meaningful error messages and better handle different types of exceptions.
What are the best practices for using LINQ in C#?
To use LINQ efficiently, prefer the query syntax over the method syntax, and avoid performing unnecessary operations or data transformations. Also, be mindful of the performance implications of your LINQ queries, especially when working with large datasets.
How can I avoid common issues with asynchronous programming in C#?
To avoid issues with asynchronous programming in C#, make sure you understand the proper use of the async and await keywords, and be aware of potential deadlocks and race conditions. Also, consider using the Task.Run() method to offload long-running tasks to a separate thread.
Why is it important to properly dispose of resources in C#?
Properly disposing of resources, such as database connections, file handles, and network sockets, is important to avoid resource leaks and ensure your application’s stability. Failing to do so can lead to performance issues and even crashes.